In today’s article, I will try to create a web app that uploads and displays images in Django.
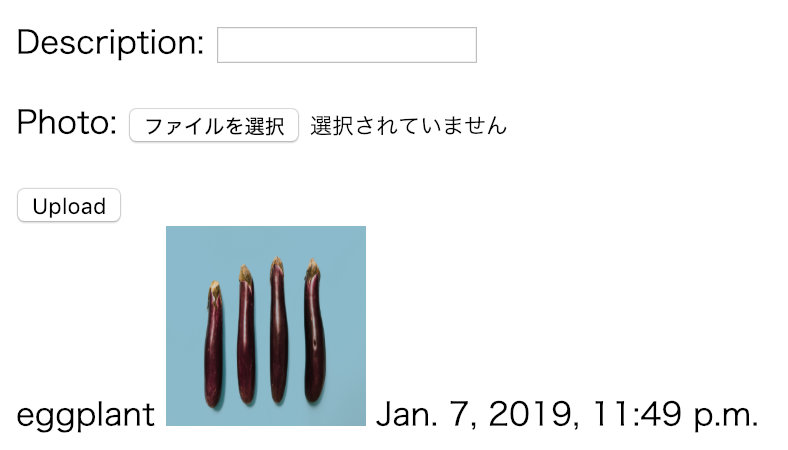
It is an application to select and upload photos. The name of the photo, the photo, and the date and time of posting are displayed.
Library installation
You need a library called pillow to handle images. Please install it with the following command.
pip install pillow
Add directory for media
Add or modify settings.py as follows.
ai_webapp/settings.py
TIME_ZONE = 'Asia/Tokyo' MEDIA_ROOT = os.path.join(BASE_DIR, 'media') MEDIA_URL = '/media/'
Create a folder named “media” under the project folder.
URL setting
Add the following settings to urls.py of the project.
ai_webapp/urls.py
from . import settings from django.contrib.staticfiles.urls import static from django.contrib.staticfiles.urls import staticfiles_urlpatterns urlpatterns = [ path('admin/', admin.site.urls), path('ai_image/', include('ai_image.urls')) ] urlpatterns += staticfiles_urlpatterns() urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Please note that without these two lines, the image will not be displayed.
model
It is a model for handling images.
from django.db import models class Document(models.Model): description = models.CharField(max_length=255, blank=True) photo = models.ImageField(upload_to='documents/', default='defo') uploaded_at = models.DateTimeField(auto_now_add=True)
By setting upload_to to ‘documents’, images will be saved in “/media/documents”.
Form
from django import forms from .models import Document class DocumentForm(forms.ModelForm): class Meta: model = Document fields = ('description', 'photo',)
View
from django.shortcuts import render, redirect from .forms import DocumentForm from .models import Document def index(request): if request.method == 'POST': form = DocumentForm(request.POST, request.FILES) if form.is_valid(): form.save() return redirect('index') else: form = DocumentForm() obj = Document.objects.all() return render(request, 'ai_image/model_form_upload.html', { 'form': form, 'obj': obj })
template
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Upload</title> </head> <body> <form method="post" enctype="multipart/form-data"> {% csrf_token %} {{ form.as_p }} <button type="submit">Upload</button> </form> {% for item in obj %} <tr> <td>{{ item.description }}</td> <td><img src="{{ item.photo.url }}" width="100" height="100"/></td> <td>{{ item.uploaded_at }}</td> <tr> {% endfor %} </body> </html>
migration
python manage.py makemigrations ai_image python manage.py migrate python manage.py runserver
Management tool
It is a method to delete the unnecessary image from the management screen.
python manage.py createsuperuser
Enter an appropriate username, email address and password.
admin.py
from django.contrib import admin from .models import Document # Register your models here. admin.site.register(Document)
You can access the management page with the following command.
http://localhost:8000/admin
Reference
https://matthiasomisore.com/web-programming/display-image-in-a-django-template-using-imagefield/